DOM(Document Object Model) - document object로 구성된 html문서 다루기
\
-
WEB3 - bootstrap
-
WEB3 - Semantic UI
-
WEB3 - React
-
WEB3 - jQuery
-
WEB3 - PHP & Oracle
-
WEB3 - PHP & Laravel
-
WEB2 - Nodejs
-
WEB3 - NodeJS & Express
-
WEB3 - NodeJS & MySQL
-
WEB3 - NodeJS & MongoDB
-
WEB2 - JSP
-
WEB2 - 광고
-
WEB2 - GIT
-
WEB2 - 결제
그자체로 문서객체로 정의
-attr(name) : 일치하는 집합에서 첫번째 엘리먼트의 속성값을 얻음
-removeAttr
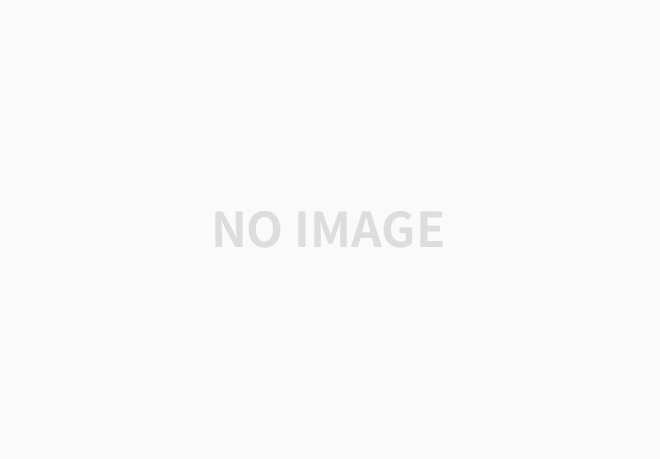
<부모 자식관계>
-append : 가장 막내로 붙인다. 부모.append(자식)
-appendTo : 자식.appendTo(부모) - 부모 밑에 자식이 들어오는 것은 동일(체인을 편리하게 걸기 위해 이렇게 표시)
-prepend : 가장 첫째로 붙인다. 부모.prepend(자식)
-prependTo : 자식.prepentTo(부모)
<형제관계>
-before : 새로운 형이 내 앞에 옴. OLD형제.before(NEW형제)
-insertBefore : NEW형제.inseftBefore(OLD형제)
-after : 새로운 동생이 내 뒤에 옴. OLD형제.after(NEW형제)
-insertAfter : NEW형제.insertAfter(OLD형제)
<chapter4-dom1>
<script src="jquery.js" type="text/javascript"></script>
<script type="text/javascript">
$(function() {
$('div').append($('b'));//부모(div)안 끝에 자식(b)을 붙임
$('span').prependTo($('b')); //부모(d)안 맨 처음에 자식('span')붙임
$('div').before($('b'));//기존형제.before('새로운형제')형제관계
$('b').insertBefore($('span'));//새로운형제.insertBefore('기존형제')
$('b').insertAfter($('div'));//위의 것과 같은 위치
alert($('body').html());
})
</script>
<chapter5>
$(function(){
$('<ol id="notes"></ol>').insertBefore('#footer');
$('span.footnote').each(function(index){//집합인데 각각의 설정을 해줘야 함 : each
$(this).before('<a id="context-'+(index+1)+'">['+(index+1)+']</a>')//this : 각각의 span, 안의 속성값은 ""
.appendTo('#notes')
.append(' <a href="#context-'+(index+1)+'">context-'+(index+1)+'</a>')
.wrap('<li></li>');
});
//ol(#notes)를 footer위에다 형제관계로 삽입
//span(.footnote) 각각을 가지고 와서 형제관계로 이 앞에 a(#context-i)생성
//ol(#notes)의 안에 맨끝에 이 span을 각각 붙임 이때 앞에 붙인 a(#context-i)는 그대로 둠
//이 span(.footnote)의 안의 맨끝에 아까 붙였던 a(#context-i)를 붙임
//ol안에는 li들어가고 그 안에 span이나 a들어와야 하니까 wrap으로 감싸줌 ol>li>a
});
<gallery>
$(function() {
$('#navi a').on('click',function(event){
$('#main img').before('<img src="'+ $(this).attr('href')+'"/>'); //새로운 이미지가 부모앞에 옴
$('#main img:last').fadeOut('slow',function(){//부모 이미지 사라짐
$(this).remove();
});
event.preventDefault();
});
});
<gallery2>
$(function() {
$('img.next').on('click',function(event){
$('.pageWrap').animate({
marginLeft : parseInt($('.pageWrap').css('marginLeft'))-300;
});
});
$('img.prev').on('click',function(event){
$('.pageWrap').animate({
marginLeft : parseInt($('.pageWrap').css('marginLeft'))+300;
});
});
});
AJAX : 즉각적인 비동기로 서버에서 값을 받아 한페이지 내에서 바로 사용가능
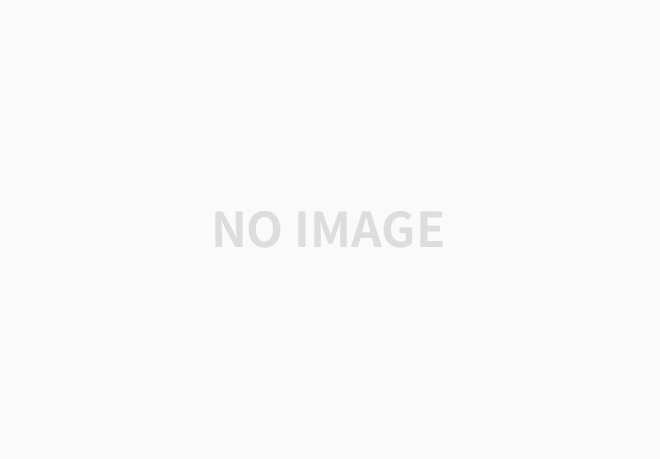
-개별함수 ( 서버가 어떤 값을 전달해주느냐에 따라)
-load(url, parameter, callback) : html결과값일 경우 사용 용이
-$get(url, parameter, callback) : XML결과값일 경우 사용 ->html로 변환해줘야 함
-$post(url, parameters, callback) : XML결과값일 경우 사용 ->html로 변환해줘야 함
-$.getJSON(url, parameters, callback) : JSON결과값일 경우 -> html로 변환해줘야 함
-통합함수
*Chapter6
-서버의 데이터타입이 다 다름
//step1 (html -> html)
$(function(){
$('#letter-a a').click(function(){
$('#dictionary').hide().load('a.html',function(){
$(this).fadeIn();
});
return false; //기본 이벤트 취소(=event.preventDefault())
});
});
//step2(json ->html)
$(function(){
$('#letter-b a').click(function(){
$.getJSON('b.json',function(data){//서버로부터 결과값을 가져오면 콜백함수 호출됨
//배열에 있는 각각의 요소를 데려와야 함
$('#dictionary').empty();
$.each(data, function(idx, item){ //item은 하나의{}뭉텅이
var html = '<div class="entry">'; //json -> html로 변경해야함
html += '<h3 class="term">'+item.term + '</h3>';
html += '<div class="part">'+item.part + '</div>';
html += '<div class ="definition">'+ item.definition +'</div>';
html += '</div>';
$('#dictionary').append(html);//부모에 맨 뒤에 붙임
});
});
return false;
});
});
ajax01 ->ajax_json.html
$(function(){
$.getJSON('ajax01.jsp',function(data){//서버로부터 결과값을 가져오면 콜백함수 호출됨
$('#treeData').append('<tr><td>이름</td><td>주소</td></tr>');
$.each(data, function(idx, item){ //item은 하나의{}뭉텅이
var html = '<tr><td>'+item.name + '</td><td>'+item.address+'</td></tr>';
$('#treeData').append(html);
});
});
});
//step3(js->html)
$(function(){
$('#letter-c a').click(function(){
$('#dictionary').empty();
$.getScript('c.js'); //자바스크립트 형태
return false; //기본 이벤트 취소(=event.preventDefault())
});
});
//step4(xml -> html)
$(function(){
$('#letter-d a').click(function(){
$('#dictionary').empty();
$.get('d.xml',function(data){
$(data).find('entry').each(function(index){
$entry = $(this); //코드의 가독성이 좋음
var html = '<div class="entry">';
html += '<h3 class="term">'+$entry.attr('term') + '</h3>';
html += '<div class="part">'+$entry.attr('part') + '</div>';
html += '<div class ="definition">'+ $entry.find('definition').text() +'</div>';
html += '</div>';
$('#dictionary').append(html);
});
});
return false; //기본 이벤트 취소(=event.preventDefault())
});
});
//step5
$(function(){
$('#letter-e a').click(function(){
$('#dictionary').empty();
$.get('server3.jsp',{'term':$(this).text()},function(data){
$('#dictionary').text(data);
});
return false; //기본 이벤트 취소(=event.preventDefault())
});
});
'FULLSTACK > WEB' 카테고리의 다른 글
WEB PROJECT (0) | 2020.11.13 |
---|---|
JQUERY 4차시 - AJAX, plug-in (0) | 2020.11.13 |
JQUERY 2차시 - each메소드, 이벤트, 스타일 (0) | 2020.11.13 |
JAVA SCRIPT 5차시, JQEURY 1차시 - Ajax (0) | 2020.11.13 |
JAVA SCRIPT 4차시 - event, this, DOM (0) | 2020.11.13 |