**재귀함수를 이용한 버블정렬
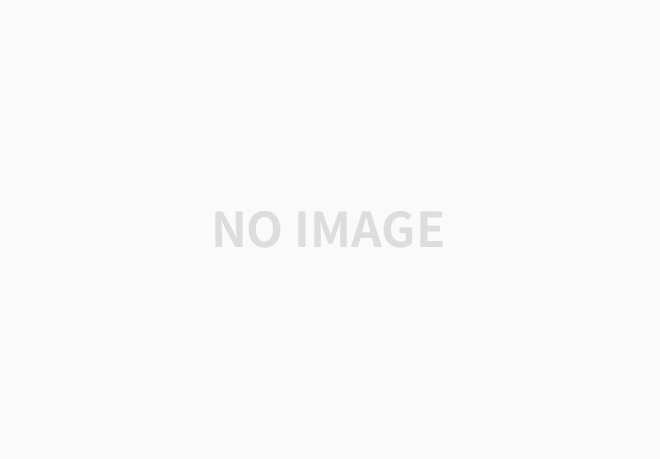
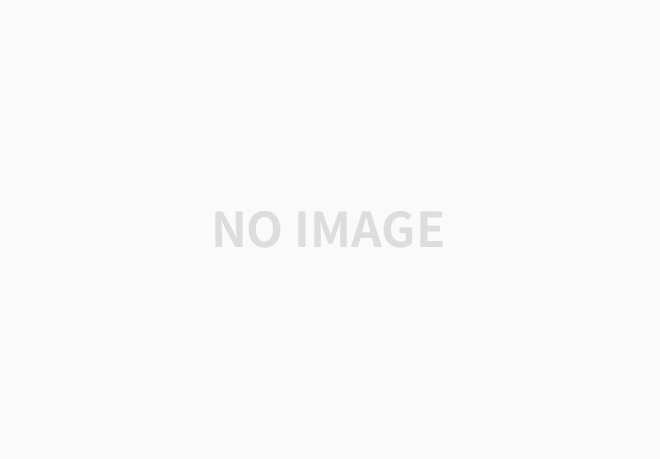
public class BubbleSort {
public static void bubbleSort(int arr[],int last) {//외부for문
if(last>0) { //재귀함수는 종료조건이 있어야 함
for(int i=1;i<=last;i++) {
if(arr[i-1]>arr[i])
swap(arr,i-1,i);
}
bubbleSort(arr, last-1);
}
}//for문 두개돌릴것을 외부for->메소드, 내부for를 재귀함수로 만들어버림.
public static void swap(int arr[],int index1, int index2) {
int temp = arr[index1];
arr[index1] = arr[index2];
arr[index2] = temp;
}
public static void printArray(int arr[]) {
for(int data : arr) {
System.out.print(data +", ");
}System.out.println();
}
public static void main(String[] args) {
int arr[]= {3,6,2,8,4,1};
printArray(arr);
bubbleSort(arr, arr.length-1);
printArray(arr);
}
}
데이터타입 -> 서로형변환 가능
object(객체)타입도 데이터타입 casting이 안됨.
->object타입도 데이터타입처럼 casting이 되었음 좋겠다.
list.add(object)넣으면 부모 자식간에 casting 가능
int -> double
자식 -> 부모로 casting
부모 -> 자식
Customer[] arr= {new VIP(),
new general(),
new 진상()}; //상속받은 것들은 부모의 배열안에 넣을 수 있다. //자식은 부모의 타입으로 형변환 될 수 있다.
메소드 오버로딩 : 메소드 내용 동일, 파라미터 값만 다름
★메소드 오버라이딩
부모내용을 상속받지만 메서드 내용을 재정의해서 사용함.
★자바의 다형성 조건
-
상속관계의 casting이 가능해야 한다.
-
부모자식간의 오버라이딩된 메소드가 있어야 한다.
메소드의 시그니처(리턴,이름,파라미터)는 같고 내용만 다르다 -> 메소드 오버라이딩
Source - override ->시그니처만 정의해줌. 업로드해줌
--account에 상속받아 MinusAccount클래스 작성하기
package Kosta.oop2;
public class MinusAccount extends Account {
private int creditLine;
public MinusAccount() {}
public MinusAccount(String ownerName, String accountNo, double balance, int creditLine) {
super(ownerName, accountNo, balance);
this.creditLine = creditLine;
}
@Override
public int withdraw(int amount) throws Exception {
if(balance + creditLine<amount) {
throw new Exception("잔액 부족");
}
balance -= amount;
return amount;
}
}
package Kosta.oop2;
public class MinusAccountMain {
public static void main(String[] args) {
MinusAccount ma =
new MinusAccount("1111","홍길동",10000,10000);
try {
ma.withdraw(15000); //error뜨면 ->부모 withdraw메소드 호출
//자식에 있는 오버라이딩 된 withdraw 우선으로 호출되어짐
} catch (Exception e) {
e.printStackTrace();
}
ma.print();
}
}
--GeneralMember에 상속받은 SpecialMember클래스 작성하기
public class SpecialMember extends GeneralMember {
private int bonus;
public SpecialMember (){}
public SpecialMember(String ID, String name, String address, int bonus) {
super(ID, name, address);
this.bonus =bonus ;
}
@Override
public void printmember() {
super.printmember();
System.out.println("회원의 보너스 포인트 : "+ bonus);
}
}
public class Main {
public static void main(String[] args) {
Video[] vrr = {
new Video("1","트랜스포머3","서봉수"),
new Video("2","쿵더팬더2","지성민")
};
GeneralMember m[] = {
new GeneralMember("aaa","홍길동","동탄"),
new SpecialMember("bbb", "김철수", "서울", 10)
};
for(int i=0;i<m.length;i++) { //video2개 m2개라 가능
m[i].setBorrowvideo(vrr[i]);
m[i].printmember();
System.out.println();
}
}
}
7장. 레퍼런스타입
-기본형 데이터타입(8가지) : 실제값이 들어감.
-나머지는 다 레퍼런스타입 : 주소값이 들어가있음.
obj1 = new Point(10, 20); -> 주소값이 들어있음.
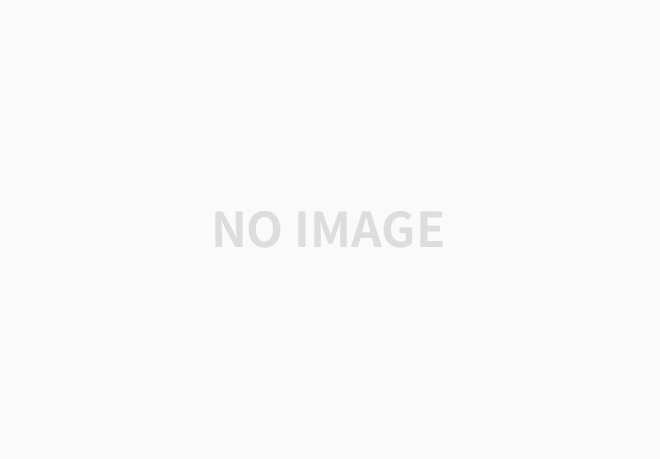
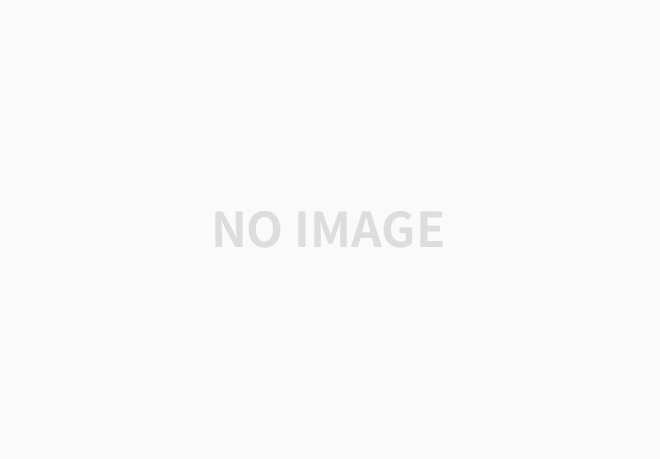
위랑 아래랑 똑같다. 파라미터로 던져주고 받은 것임. 주소값 같은 곳 참조.
자식->부모 형변환 : 자동형변환
자식만이 가지고 있는 변수나 메소드는 더이상 호출이 불가능.
부모로 부터 상속된 것과 오버라이딩 된 메소드만 호출 가능
형변환해도 오버라이딩된 메소드는 접근가능
같은 공통의 메소드나 변수를 사용하기 위해서
부모->자식 형변환 : 강제형변환 (데이터손실 X) - 원래 자식이 부모로 갔다가 자식으로 갔을때만 가능
double -> int : 강제 형변환
부모를 자식의 데이터타입으로 가야겠다 하면 강제 형변환을 해줘야 함.
자식이 원래 가지고있던 메소드나 변수를 사용하기 위해서
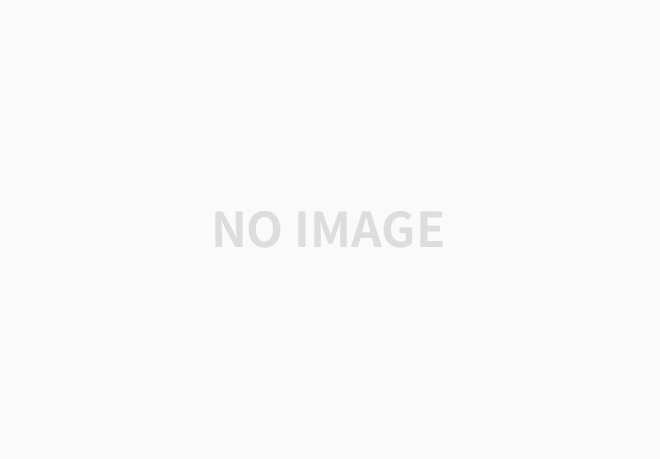
※원래 부모였다가 자식으로 갔다가 부모로 다시 돌아오면 강제 형변환 에러뜸. (casting exception)
※자식에게만 있는 메소드를 사용하기 위해서는 강제 형변환 다시해줘야 함.
그래서 나온 것이 instanceof 연산자
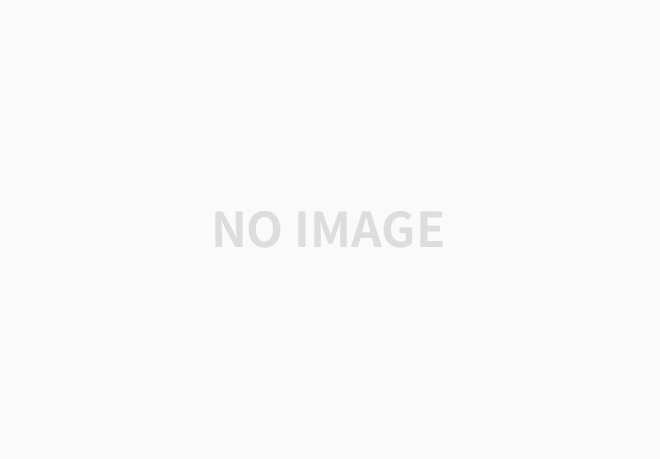
형변환 가능 = True
형변환 불가능 = False
<Phoneinfo응용문제>
Main, Manager
Phoneinfo배열 안에 넣기- Universe(University인데 내가 잘못침), Company
public void addPhoneinfo()
//1.일반 2.동창(major, year) 3.직장(dept, position)
public void listPhoneinfo()
//1.전체 2.동창(major, year) 3.직장(dept, position)
-Manager클래스
public class Manager {
Phoneinfo p[];
Scanner sc = new Scanner(System.in);
int count = 0; //지역변수
public Manager() {
p = new Phoneinfo[10];
} //default 생성자
public void addPhoneinfo() {
System.out.print("1.일반 2.동창(major, year) 3.직장(dept, position) : ");
String num = sc.nextLine();
System.out.print("이름 : ");
String name= sc.nextLine();
System.out.print("전화번호 : ");
String phoneNo= sc.nextLine();
System.out.print("생년월일 : ");
String birth= sc.nextLine();
switch (num) {
case "1":
p[count++] = new Phoneinfo(name, phoneNo, birth);
break;
case "2" :
System.out.print("출신학과 : ");
String major= sc.nextLine();
System.out.print("출신학번 : ");
String year= sc.nextLine();
p[count++] = new Universe(name, phoneNo, birth, major, year);//phoneinfo객체배열안에 universe 들어갈 수 있음. : 자식->부모 형변환(자동형변환)
break;
case "3":
System.out.print("회사부서 : ");
String dept= sc.nextLine();
System.out.print("회사직급 : ");
String position= sc.nextLine();
p[count++] = new Company(name, phoneNo, birth, dept, position); : 자식->부모 형변환(자동형변환)
break;
}
}
-Manager클래스 안의 listPhoneinfo메소드
public void listPhoneinfo() {
//배열에 있는 모든 Phoneinfo객체 출력
//show()출력
//1.전체 2.동창(major, year) 3.직장(dept, position)
//for문과 instanceof를 사용하여 각각 맞는 답을 찾아서 연결해줌
System.out.print("1.전체 2.동창(major, year) 3.직장(dept, position) : ");
String num = sc.nextLine();
//강사님코드
for(int i=0;i<count;i++) {
if(num.equals("1")&&p[i] instanceof Phoneinfo)
p[i].show();
if(num.equals("2")&&p[i] instanceof Universe)
p[i].show();
else if(num.equals("3")&&p[i] instanceof Company)
p[i].show();
}
switch(num) {
case "1" :
for(int i=0;i<count;i++) {
if(p[i] instanceof Phoneinfo)
p[i].show();
}
break;
case "2" :
for(int i=0;i<count;i++) {
if(p[i] instanceof Universe)
p[i].show();
}
break;
case "3" :
for(int i=0;i<count;i++) {
if(p[i] instanceof Company)
p[i].show();
}
break;
}
}
public class Universe extends Phoneinfo {
private String major;
private String year;
public Universe(String name, String phoneNo, String birth, String major, String year) {
super(name, phoneNo, birth);
this.major = major;
this.year = year;
}
public Universe() {}
public void show2() {
System.out.println(major+ " : "+year);
}
@Override
public void show() {
// TODO Auto-generated method stub
super.show();
System.out.println("출신학과 : "+major);
System.out.println("출신학번 : "+year);
}
@Override
public String getName() {
// TODO Auto-generated method stub
return super.getName();
}
@Override
public String getPhoneNo() {
// TODO Auto-generated method stub
return super.getPhoneNo();
}
@Override
public String getBirth() {
// TODO Auto-generated method stub
return super.getBirth();
}
}
public class Company extends Phoneinfo {
private String dept;
private String position;
public Company(String name, String phoneNo, String birth, String dept, String position) {
super(name, phoneNo, birth);
this.dept = dept;
this.position = position;
}
public Company() {}
@Override
public void show() {
// TODO Auto-generated method stub
super.show();
System.out.println("회사부서 : "+dept);
System.out.println("회사직급 : "+position);
}
@Override
public String getName() {
// TODO Auto-generated method stub
return super.getName();
}
@Override
public String getPhoneNo() {
// TODO Auto-generated method stub
return super.getPhoneNo();
}
@Override
public String getBirth() {
// TODO Auto-generated method stub
return super.getBirth();
}
}
******형변환
for(int i=0;i<count;i++) {
if(num.equals("1")&&p[i] instanceof Phoneinfo)
p[i].show();
if(num.equals("2")&&p[i] instanceof Universe) {
p[i].show();
Universe uni = (Universe)p[i]; 자식이 자신고유의 메소드를 사용하기 위해 자식->부모->자식 형변환(강제형변환)
uni.show2();
}
else if(num.equals("3")&&p[i] instanceof Company)
p[i].show();
}
Mission
오늘 배운것 할 수 있는 예제 하나씩 만들어보기. 각자 Design해보기
확장할 수 있는 자식클래스, 강제형변환, 상속
-tag클래스
동물저장소에 동물정보를 저장
-status클래스
건강정보 출력
Mom(name, age, weight)
puppy- mom으로부터 상속받음(antibody, health)
Manage
Main 1. 반려견 등록 2. 출력 3. 수정 4.검색(동일견종으로 검색) 5. 건강상태 진단 6. 종료