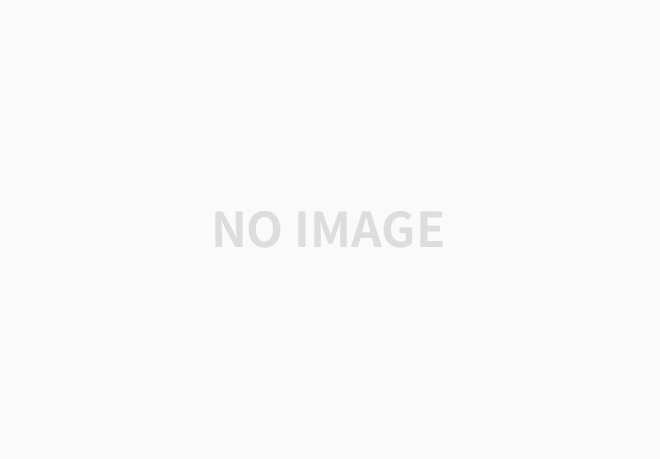
☺️😊☺️😘😭😩💕🥰🥸🥺🥱
--blog(app)
-
테이블 설계
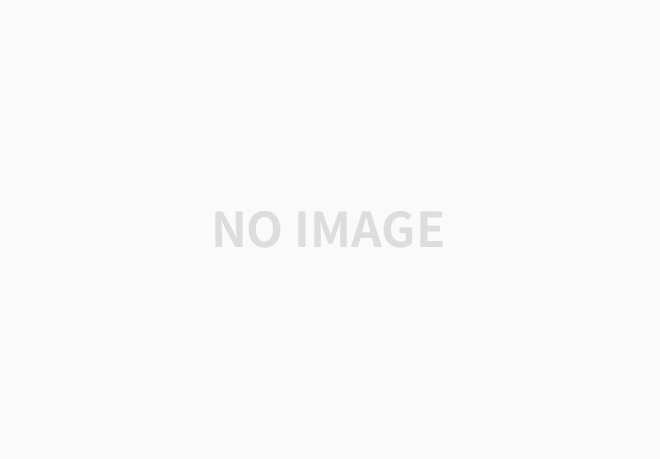
-
URL 설계
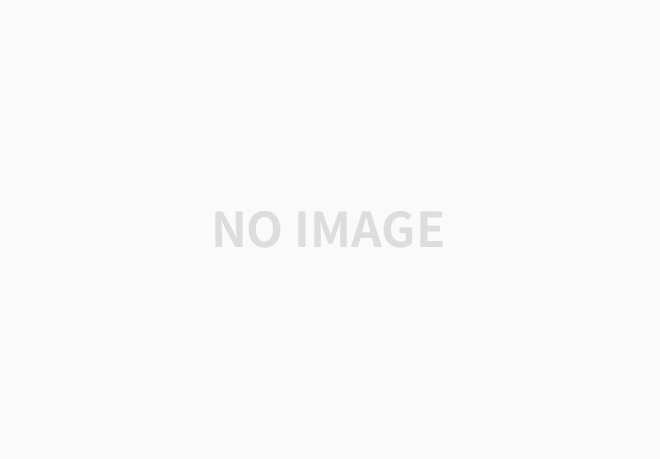
https://blog.gaerae.com/2016/11/what-is-library-and-framework-and-architecture-and-platform.html
[개발용어] 라이브러리, 프레임워크, 아키텍처, 플래폼이란?
개발 입문자나 혹은 현업 개발자이지만 정의를 내리기 곤란한 라이브러리, 프레임워크, 아키텍처, 플랫폼에 대한 개인적인 생각을 정리했습니다.
blog.gaerae.com
**API, Library, Platform, Framework이 각각 무엇인지 찾아보기
-
API(Application Programming Interface, 응용 프로그램 프로그래밍 인터페이스)는 응용 프로그램에서 사용할 수 있도록, 운영 체제나 프로그래밍 언어가 제공하는 기능을 제어할 수 있게 만든 인터페이스를 뜻한다.
-
Library : 재사용이 필요한 기능으로 반복적인 코드 작성을 없애기 위해 언제든지 필요한 곳에서 호출하여 사용할 수 있도록 Class나 Function으로 만들어진 것이다. 사용 여부는 코드 작성자 선택 사항이며 새로운 라이브러리 제작 시에도 엄격한 규칙이 존재하지 않는다. 제작 의도에 맞게 작성.
-
Platform : 프로그램이 실행되는 환경이며 플랫폼은 플랫폼위에 다른 플랫폼이 존재할 수 있다. 가령, Windows에서 Java로 개발하고 있으며 앱스토어에서 어플을 내려받는 과정에서 이미 3개의 플랫폼을 사용하고 있는 것이다. 플랫폼은 같은 영역에도 다양한 목적과 가치로 많이 만들어지고 있으며 모든 플랫폼에서 실행되도록 개발하기는 어렵다. 프로그램의 목적에 맞도록 플랫폼을 선택하는 것이 중요하다.
-
Framework : 원하는 기능 구현에만 집중하여 빠르게 개발 할 수 있도록 기본적으로 필요한 기능을 갖추고 있는 것으로 위에서 설명한 라이브러리가 포함되어 있다.프레임워크만으로는 실행되지 않으며 기능 추가를 해야 되고 프레임워크에 의존하여 개발해야 되며 프레임워크가 정의한 규칙을 준수해야 한다.
-
Architecture : 기획한 내용을 프로그램화했을 경우 필요한 주요 특징을 기술적으로 설계하고 명시하는 것이다. 결과물에 필요한 모든 구성 요소를 명시하지만, 구체적인 구현 방법은 포함되어 있지 않다. 가령, 아래에서 설명할 플랫폼은 주요 특징이지만 프레임워크와 라이브러리는 주요 특징이 아니므로 명시되지 않을 가능성이 크다.
**작업/코딩 순서
-
테이블 정의에 관련된 Model 코딩
-
URLconf
-
뷰 작성
-
템플릿 작성
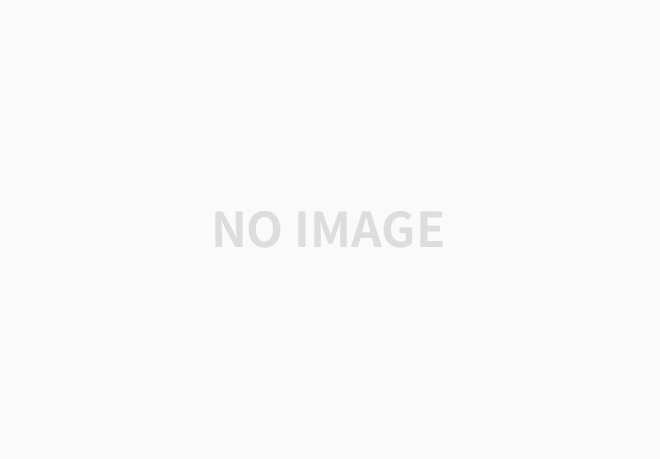
#settings.py
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'bookmark.apps.BookmarkConfig',
'blog.apps.BlogConfig',
]
#blog/models.py
from django.db import models
# Create your models here.
# print() -> {{}}
class Post(models.Model):
title = models.CharField('TITLE', max_length=50)
slug = models.SlugField('SLUG', unique=True, allow_unicode=True, help_text='one word for this alias')
description = models.CharField('DESCRIPTION', max_length=100, blank=True, help_text='simple description text')
content = models.TextField('CONTENT')
create_date = models.DateTimeField('Create Date', auto_now_add=True)
modify_date = models.DateTimeField('Modify Date', auto_now=True)
def __str__(self):
return self.title
class Meta:
verbose_name = 'post'
verbose_name_plural = 'posts'
db_table = 'blog_posts'
# ordering = ('modify_date', 'author') # asc & author
ordering = ('-modify_date',) # desc,
def get_absolute_url(self):
return reverse('blog:post_detail', args=(self.slug,))
# http://127.0.0.1:8000/blog/post/daily 튜플형태 사용은 ( ,) 콤마 붙여주기
def get_previous_post(self):
return self.get_previous_by_modify_date()
def get_next_post(self):
return self.get_next_by_modify_date()
**ListView, DetailView 참고
https://docs.djangoproject.com/en/3.1/ref/models/instances
Model instance reference | Django documentation | Django
Django The web framework for perfectionists with deadlines. Overview Download Documentation News Community Code Issues About ♥ Donate
docs.djangoproject.com
-
Data Field 종류
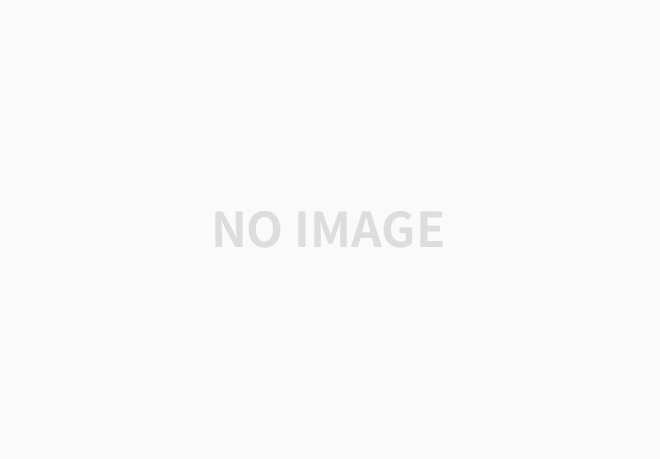
#blog/admin.py
from django.contrib import admin
from blog.models import Post
# Register your models here.
class PostAdmin(admin.ModelAdmin):
list_display = ('title', 'modify_date')
list_filter = ('modify_date')
search_fields = ('title', 'content')
prepopulated_fields = {'slug' : ('title',)}
admin.site.register(Post, PostAdmin)
$python manage.py makemigrations
$python manage.py migrate
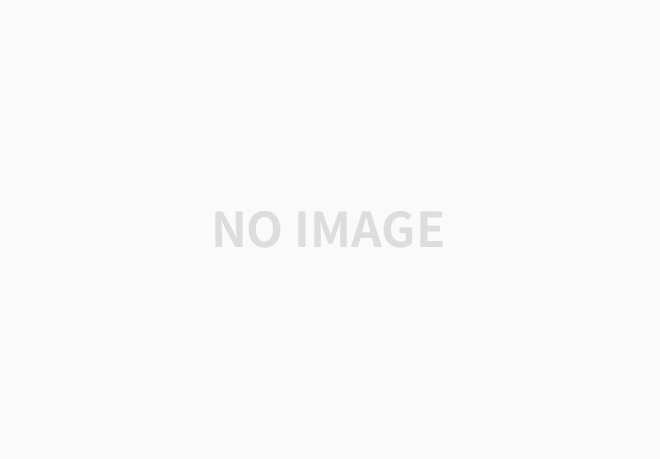
#mysite/urls.py
from django.contrib import admin
from django.urls import path, include
from bookmark.views import BookmarkLV, BookmarkDV
urlpatterns = [
path('admin/', admin.site.urls),
path('bookmark/', include('bookmark.urls'), namespace='bookmark'),
path('blog/', include('blog.urls'), namespace='blog'),
]
#bookmark/urls.py
from django.contrib import admin
from django.urls import path, include
from bookmark.views import BookmarkLV, BookmarkDV
app_name = 'bookmark'
urlpatterns = [
path('', BookmarkLV.as_view(), name='index'),
path('<int:pk>', BookmarkDV.as_view(), name='detail'),
]
#blog/urls.py
from django.urls import path
from blog.views import *
app_name = 'blog'
urlpatterns = [
# /blog/
path('', PostLV.as_view(), name='post_list'),
# /blog/post/
path('post/', PostLV.as_view(), name='post_list'),
# /blog/post/python-programming
path('post/<str:slug>', PostDV.as_view(), name='post_detail'),
# /blog/archive
path('archive/', PostAV.as_view(), name='post_archieve'),
# /blog/2020
path('<int:year>/', PostYAV.as_view(), name='post_year_archive'),
# /blog/2020/01
path('<int:year>/<str:month>/', PostMAV.as_view(), name='post_month_archieve'),
# /blog/2020/01/20
path('<int:year>/<str:month>/<int:day>/', PostDAV.as_view(), name='post_day_archive'),
# /blog/today
path('today/', PostTAV.as_view(), name='post_today_archive'),
]
**YearArchiveView 참고
https://docs.djangoproject.com/en/3.1/ref/class-based-views/generic-date-based
Generic date views | Django documentation | Django
Django The web framework for perfectionists with deadlines. Overview Download Documentation News Community Code Issues About ♥ Donate
docs.djangoproject.com
#blog/views.py
from django.views.generic import ListView, DetailView
from django.views.generic.dates import ArchiveIndexView, YearArchiveView, MonthArchiveView, DayArchiveView, TodayArchiveView
from blog.models import Post
# Create your views here.
class PostLV(ListView):
model = Post
template_name = "blog/post_all.html"
context_object_name = "posts" # object_list
paginate_by = 3
class PostDV(DetailView):
model = Post
class PostAV(ArchiveIndexView):
model = Post
date_field = "modify_date"
class PostYAV(YearArchiveView):
model = Post
make_object_list = True
date_field = "modify_date"
class PostMAV(MonthArchiveView):
model = Post
date_field = "modify_date"
class PostDAV(DayArchiveView):
model = Post
date_field = "modify_date"
class PostTAV(TodayArchiveView):
model = Post
date_field = "modify_date"
**오류 잡기(url설정)
->model에서 정의 : reverse()가 해줌
->나중에 웹퍼블리셔가 정리하기 편한 방식
def get_absolute_url(self):
return reverse('blog:post_detail', args=(self.slug,))
<li><a href="{{ post.get_absolute_url }}">{{ post }}</a></li>
->template에서 정의 : url이 해줌
<li><a href="{% url 'blog:post_detail' post.slug %}">{{ post }}</a></li>
#blog/templates/post_all.html
[
{{ page_obj }}
{{ page_obj.has_previous }}
{{ page_obj.has_next}}
]
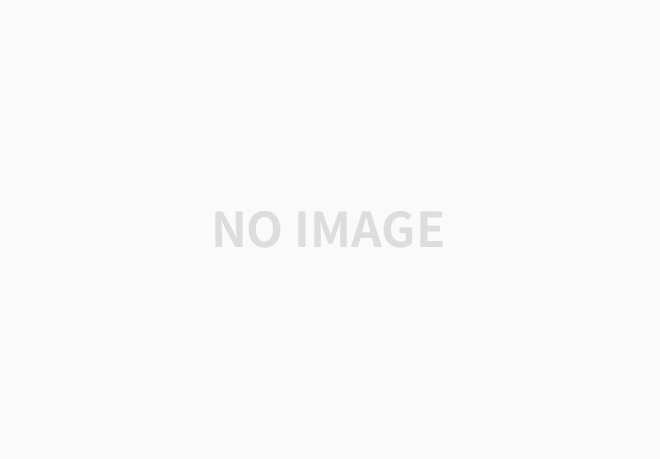
<h1>Blog List</h1>
<!-- display blog list -->
<ul>
{% for post in posts %}
<h2><a href='{{ post.get_absolute_url }}'>{{ post.title }}</a></h2>
{{ post.modify_date }}
<p>{{ post.description }}</p>
{% endfor %}
</ul>
<br/>
<!-- paging link -->
<div>
{% if page_obj.has_previous %}
<a href="?page={{ page_obj.previous_page_number }}"><<</a>
{% endif %}
[ Page {{page_obj.number}} / {{ page_obj.paginator.num_pages}} ]
{% if page_obj.has_next %}
<a href="?page={{ page_obj.next_page_number }}">>></a>
{% endif %}
</div>
*날짜 표기법 참고
https://docs.djangoproject.com/en/3.1/ref/templates/builtins
Built-in template tags and filters | Django documentation | Django
Django The web framework for perfectionists with deadlines. Overview Download Documentation News Community Code Issues About ♥ Donate
docs.djangoproject.com
#blog/templates/post_detail.html
<h2>{{ object.title }}</h2>
<!-- previous or next post link -->
<p class="other_posts">
<a href="{{ object.get_previous_post.get_absolute_url }}">{{ object.get_previous_post }}</a>
|
<a href="{{ object.get_next_post.get_absolute_url }}">{{ object.get_next_post }}</a>
</p>
<!-- date -->
<p class="date">
{{ object.modify_date | date:"Y-m-d H:i" }}
</p>
<br/>
<!-- post content -->
<div class="body">
{{ object.content | linebreaks }}
</div>
$python manage.py dbshell(작성날짜 수정하기 -> 필터링하기 위해)
sqlite>update blog_posts set modify_date=datetime(modify_date, '-3 month') where id=1;
sqlite>update blog_posts set modify_date=datetime(modify_date, '-2 month') where id=2;
sqlite>update blog_posts set modify_date=datetime(modify_date, '-1 month') where id=3;
sqlite>update blog_posts set modify_date=datetime(modify_date, '-1 month') where id=4;
sqlite> select id, modify_date from blog_posts;
1|2020-10-21 02:19:25
2|2020-11-21 02:20:18
3|2020-12-21 02:20:38
4|2020-12-21 02:53:04
5|2021-01-21 05:55:57.418229
6|2021-01-21 05:56:12.212508
7|2021-01-21 05:56:33.015180
#blog/templates/post_archive.html
<h1>Post Archives until {% now "Y m d"%}</h1>
<ul>
{% for date in date_list %}
<li style="display: inline;">
<a href="{% url 'blog:post_year_archive' date|date:'Y' %}">{{ date | date:'Y' }}</a>
</li>
{% endfor %}
</ul>
<br/>
<div>
<ul>
{% for post in object_list %}
<li>
{{ post.modify_date | date:"Y-m-d" }}
<a href='{{ post.get_absolute_url }}'><strong>{{ post.title }}</strong></a>
</li>
{% endfor %}
</ul>
</div>
#blog/templates/post_archive_year.html
<h1>Post Archives for {{ year|date:'Y' }}</h1>
<ul>
{% for date in date_list %}
<li style="display: inline;">
<a href="{% url 'blog:post_month_archive' date|date:'Y' date|date:'m' %}">{{ date | date:'F' }}</a>
</li>
{% endfor %}
</ul>
<br/>
<div>
<ul>
{% for post in object_list %}
<li>
{{ post.modify_date | date:"Y-m-d" }}
<a href='{{ post.get_absolute_url }}'><strong>{{ post.title }}</strong></a>
</li>
{% endfor %}
</ul>
</div>
#blog/templates/post_archive_month.html
<h1>Post Archives for {{ month|date:'Y, N' }}</h1>
<ul>
{% for date in date_list %}
<li style="display: inline;">
<a href="{% url 'blog:post_day_archive' date|date:'Y' date|date:'m' date|date:'d' %}">{{ date | date:'d' }}</a>
</li>
{% endfor %}
</ul>
<br/>
<div>
<ul>
{% for post in object_list %}
<li>
{{ post.modify_date | date:"Y-m-d" }}
<a href='{{ post.get_absolute_url }}'><strong>{{ post.title }}</strong></a>
</li>
{% endfor %}
</ul>
</div>
#blog/templates/post_archive_day.html
<h1>Post Archives for {{ day|date:'Y, N, d'}}</h1>
<div>
<ul>
{% for post in object_list %}
<li>
{{ post.modify_date | date:"Y-m-d" }}
<a href='{{ post.get_absolute_url }}'><strong>{{ post.title }}</strong></a>
</li>
{% endfor %}
</ul>
</div>
**TodayArchiveView -> dayArchiveView를 참조하기 때문에 post_archive_day와 연동됨.
https://docs.djangoproject.com/en/3.1/ref/class-based-views/generic-date-based
Generic date views | Django documentation | Django
Django The web framework for perfectionists with deadlines. Overview Download Documentation News Community Code Issues About ♥ Donate
docs.djangoproject.com
class TodayArchiveView¶
A day archive page showing all objects for today. This is exactly the same as django.views.generic.dates.DayArchiveView, except today’s date is used instead of the year/month/day arguments.
'CLOUD > Django' 카테고리의 다른 글
1/25 Django - 복습(RESTful API) (0) | 2021.01.25 |
---|---|
1/22 Django - 복습(Templates, Static , Search with VS Code) (0) | 2021.01.22 |
1/20 - Django 복습(with VS Code) (0) | 2021.01.20 |
1/15 Django 4차시 - blog app 만들기(Maria DB, 페이징처리, django REST frame) (0) | 2021.01.15 |
1/14 Django 3차시 - blog app만들기(CRUD + 로그인/로그아웃 + reply) (0) | 2021.01.14 |