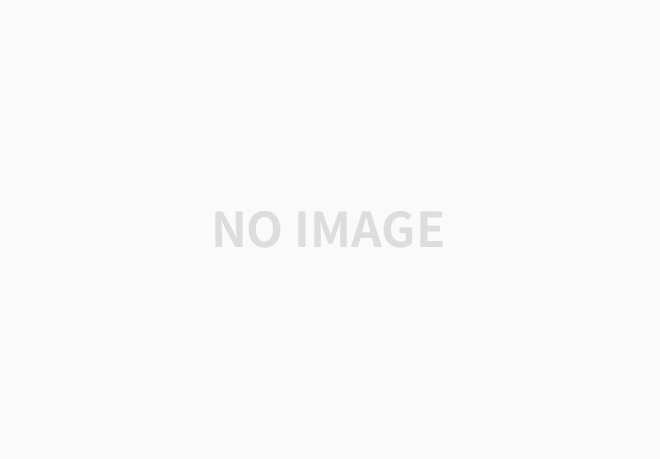
-복습 Test1
//최대값, 최소값 구하기
int score[] = {23,65,15,39,93,26,44};
int max, min = 0;
max=score[0];
min=score[0];
for(int i=1;i<score.length;i++) {
if(score[i]>max)
max = score[i];
else if(score[i]<min)
min = score[i];
}
System.out.println("최대값은 :" + max);
System.out.println("최소값은 :" + min);
//스왑 구현 (12 <==>34)
int a = 12;
int b= 34;
int tem;
tem = a;
a=b;
b=tem;
System.out.println("a= " +a);
System.out.println("b= " +b);
-random은 double형 값을 return해줌. -> 형변환을 해줘야 함 int로
-
0.0 <= Math.random() < 1.0
//난수 구하기-방법1
int n = ((int)Math.random()*6)+1;//(0~5)+1 : 1~6까지의 숫자
System.out.println(n);
//난수 구하기-방법2
Random i = new Random();
int n2 = i.nextInt(6);//0~5까지 나옴
System.out.println(n2);
Arrays.toString(numArr) = 배열 -> 문자열로 변환
//random으로 섞기
int score[] = {23,65,15,39,93,26,44};
System.out.print(Arrays.toString(score));
for(int i=0;i<100;i++) {
int n= (int)(Math.random()*7);//배열에서 0~6까지의 난수발생
int tmp = score[0];
score[0] = score[n];
score[n] = tmp;
}
System.out.println(Arrays.toString(score));
>>>{26,44,23,93,65,15,39}
-중첩구문
for(int i=1;i<=3;i++) {
for(int j=1;j<=2;j++) {
System.out.println("i=" + i+"j= "+j);
}
}
i=1j= 1
i=1j= 2
i=2j= 1
i=2j= 2
i=3j= 1
i=3j= 2
//구구단 2~9단 출력
for(int i=2;i<=9;i++) {
System.out.println("<"+i+"단>");
for(int j=1;j<=9;j++) {
System.out.println(i+"*"+j+"="+i*j);
}
}
<2단>
2*1=2
2*2=4
2*3=6
2*4=8
2*5=10
2*6=12
2*7=14
2*8=16
2*9=18
....
//4x+5y = 60
for(int x=0;x<=15;x++) {
for(int y=0;y<=12;y++) {
if(4*x+5*y==60)
System.out.println("x= "+x+"y= "+y);
}
}
x= 0y= 12
x= 5y= 8
x= 10y= 4
x= 15y= 0
//*
//**
//***
//****
//*****
for(int i=1;i<=5;i++) {
for(int j=1;j<=i;j++) {
System.out.print("*");
}System.out.println();
}
//*****
//****
//***
//**
//*
for(int i=5;i>0;i--) {
for (int j=1;j<=i;j++){
System.out.print("*");
}System.out.println();
}
for(int i=1;i<=5;i++) {
for(int j=5;j>=i;j--) {
System.out.print("*");
}System.out.println();
}
//입력한 정수를 이용하여 피라미드 구성하기
Scanner sc = new Scanner(System.in);
System.out.print("원하는 정수를 입력해주세요: ");
int rows = sc.nextInt();
int rowcount = 1;
for(int i=rows;i>=1;i--) {
for(int j=1;j<=i;j++) {
System.out.print(" ");
}
for(int k=1;k<=rowcount; k++) {
System.out.print("△"+" ");
}
System.out.println();
rowcount++;
}
원하는 정수를 입력해주세요: 6
△
△ △
△ △ △
△ △ △ △
△ △ △ △ △
△ △ △ △ △ △
Scanner sc = new Scanner(System.in);
System.out.print("원하는 정수를 입력해주세요:");
int rows = sc.nextInt();
int rowcount = 1;
for(int i=1;i<=rows;i++) {
for(int j=5;j>=i;j--) {
System.out.print(" ");
}
for(int k=1;k<=rowcount; k++) {
System.out.print("△"+" ");
}
System.out.println();
rowcount++;
}
원하는 정수를 입력해주세요:5
△
△ △
△ △ △
△ △ △ △
△ △ △ △ △
Scanner sc = new Scanner(System.in);
System.out.print("원하는 정수를 입력해주세요:");
int rows = sc.nextInt();
for(int i=1;i<=rows;i++) {
for(int j=5;j>=i;j--) {
System.out.print(" ");
}
for(int k=1;k<=i; k++) {
System.out.print("△"+" ");
}
System.out.println();
}
원하는 정수를 입력해주세요:5
△
△ △
△ △ △
△ △ △ △
△ △ △ △ △
for(int i=0;i<5;i++) {
for(int j=0;j<5+i;j++) {
if(i+j>3) {
System.out.print("*");
}else {
System.out.print(" ");
}
}
System.out.println();
}
*
***
*****
*******
*********
-2차원 배열
int arr[][];//2차원 배열 선언
arr = new int[3][2];
//2차원 배열의 크기를 상이하게 생성
int arr2[][] = new int[3][];
arr2[0]=new int[2];
arr2[1]=new int[3];
arr2[2]=new int[4];
//2차원 배열 선언, 생성, 초기화
int arr3[][]= {{1,2},{3,4,5},{6,7,8,9}};
//중첩for문을 이용하여 2차원배열 내용을 전체 출력하시오.
for(int i=0;i<arr3.length;i++){//length는 항상 1차원 크기
for(int j=0; j<arr3[i].length;j++) {
System.out.println("arr3["+i+"]["+j+"]="+arr3[i][j]);
}
}
arr3[0][0]=1
arr3[0][1]=2
arr3[1][0]=3
arr3[1][1]=4
arr3[1][2]=5
arr3[2][0]=6
arr3[2][1]=7
arr3[2][2]=8
arr3[2][3]=9
//국영수 점수를 받아 총점,평균
//2명 이상의 국영수성적을 입력받아 => 2차원 int 배열 구현
//국어, 영어, 수학, 총점, 평균 ,\n(줄바꾸기),\t(단 띄우기)
//과목에 해당하는 1차원 배열 string
//학생 1의 국어 성적:
//학생 1의 수학 성적:
Scanner sc = new Scanner(System.in);
int arr[][]=new int[2][5];
String subject[] = {"국어", "영어", "수학", "총점", "평균"};
for(int i=0;i<arr.length;i++) {//사람(가장바깥)
for(int j=0;j<3;j++) {
System.out.print("학생 "+(i+1)+"의 "+subject[j]+"점수를 입력해주세요: ");
arr[i][j]=sc.nextInt();
arr[i][3] += arr[i][j];
}
arr[i][4] = arr[i][3]/3;
}
//과목
for(String s: subject) {//향상된 for문
System.out.print(s+ "\t");
}
System.out.println();
for(int i=0; i<arr.length;i++) {
for(int j=0;j<arr[i].length;j++) {
System.out.print(arr[i][j]+"\t");
}
System.out.println();
}
학생 1의 국어점수를 입력해주세요: 60
학생 1의 영어점수를 입력해주세요: 60
학생 1의 수학점수를 입력해주세요: 60
학생 2의 국어점수를 입력해주세요: 60
학생 2의 영어점수를 입력해주세요: 60
학생 2의 수학점수를 입력해주세요: 60
국어 영어 수학 총점 평균
60 60 60 180 60
60 60 60 180 60
-배열의 복사(arraycopy, copyOf(),copyOfRange())
String oldArr[] = new String[3];
oldArr[0] = "10";
oldArr[1] = "20";
String newArr[] = new String[2];
System.arraycopy(oldArr, 0, newArr, 0, newArr.length);
System.out.println(Arrays.toString(newArr));
[10, 20]
★★주말과제 : Mission6,7,8
New York is 3 hours ahead of California, but that doesn't make California slow.
Someone graduated at the age of 22, but waited 5 years before securing a good job.
Someone became a CEO at 25, and died at 50. While another became a CEO at 50, and lived to 90 years.
Someone is still single, while someone else got married.
Obama retired at 55, and Trump started at 70.
Everyone in this world works based on their time zone.
People around you might seem to be ahead of you, and some might seem to be behind you.
But everyone is running their own race, in their own time.
Do not envy them and do not mock them.
They are in their time zone, and you are in yours.
Life is about waiting for the right moment to act.
So, relax.
You're not late. You're not early. You are very much on time.
'FULLSTACK > JAVA' 카테고리의 다른 글
JAVA 5차시 - Call by value, Call by reference, 유클리드 호제법(최대공약수 만들기), 재귀함수, arraySort, 선택정렬 (0) | 2020.11.12 |
---|---|
JAVA 주말과제 및 복습 - Mission 6,7,8 & 중복for문 연습 (0) | 2020.11.12 |
JAVA 3차시 - IF, SWITCH, WHILE, FOR, 1차배열 (0) | 2020.11.12 |
JAVA 2차시 - 변수설정, 연산자, IF문, Switch문 (0) | 2020.11.12 |
JAVA 1차시 - OT&자바실행키&형변환 (0) | 2020.11.12 |