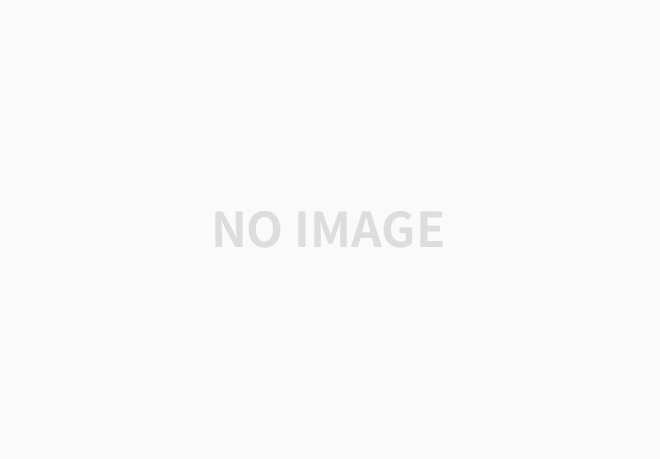
list자료구조 - 배열처럼 순서가 있다.
ArrayList<String> list = new ArrayList<String>();
list.add("포도");
List : 자료의 순서가 있다, 중복허용 (87~88%)
Map : 데이터가 쌍으로 있음. 데이터의 순서가 없음. (10%)
Set : 데이터의 순서가 없음. 중복된 데이터 값 허용x (2~3%)
ex)로또번호추출
set -> 중복값 허용x, 순서x
//Set 이용
Set<Integer> set = new HashSet<Integer>();
Random r = new Random();
for(int i=0; set.size()<6;i++) {
set.add(r.nextInt(45)+1);
}
System.out.println(set); //오버라이딩 toString메소드가 있기 때문에 바로출력됨.
[1, 34, 22, 26, 43, 45]
**스택
데이터를 넣은 순서의 역순으로만 꺼낼 수 있는 자료구조
스택으로 사용가능한 클래스 : LinkedList클래스
LinkedList<Integer> stack = new LinkedList<Integer>();
stack.addLast();
<push & pop>
LinkedList<Integer> stack = new LinkedList<Integer>();
stack.addLast(new Integer(12)); //(12)만쓰는것이 자동박싱.
stack.addLast(new Integer(59));
stack.addLast(new Integer(7));
while(!stack.isEmpty()) {
Integer num = stack.removeLast();
System.out.println(num);
}
7
59
12
**큐
데이터를 넣은 순서와 같은 순서로만 꺼낼 수 있는 자료구조
큐로 사용가능한 클래스 : LinkedList클래스
LinkedList<Integer> queue = new LinkedList<Integer>();
queue.offer();
queue.poll() -> 실제 데이터가 리턴되며 뽑힘.
queue.peek(); -> 실제 데이터가 리턴되지만 뽑히진 않음.
<offer&poll>
LinkedList<String> queue = new LinkedList<String>();
queue.offer("토끼");
queue.offer("사슴");
queue.offer("호랑이");
while(!queue.isEmpty()) {
String str = queue.poll();
System.out.println(str);
}
토끼
사슴
호랑이
**해쉬 테이블(구버전) -> 해쉬맵(신버전)
여러 개의 통을 만들어 두고 키 값을 이용하여 데이터를 넣을 통 번호를 계산하는 자료구조
해쉬 테이블로 사용할 수 있는 클래스 : HashMap클래스
HashMap<String, Integer> hashtable = new HashMap<String, Integer>(); //(키 값, 데이터)
Map<String, String> map =
new HashMap<String, String>();
map.put("1", "NC");
map.put("2", "두산");
map.put("3", "키움");
System.out.println("요소의 사이즈 : " +map.size());
if(map.containsValue("두산")) {
map.remove("2");
}
System.out.println("요소의 사이즈 : " +map.size());
요소의 사이즈 : 3
요소의 사이즈 : 2
Map(인터페이스).Entry(내부인터페이스) : Map안에 key, value 한 쌍을 가져올때
**안에 있는 데이터를 순회해서 출력
Iterator메소드 : 모든 자료구조를 통틀어서 사용가능 -> list와 set각각 상황에 맞게 쓰되 map에 쓰려먼 set을 불러온 다음에 사용해야 함
Iterator<String> iter = list.iterator();
while(iter.hasNext()) {
System.out.println(iter.next()+", ");
}
System.out.println();
}
//Map 전체목록 출력
//Map => Set => Iterator
Set set = map.entrySet();//key,value값을 다 가지고 오고 싶을때는 entrySet, key값만 keySet(), value값만 values()
Iterator iter = set.iterator();
while(iter.hasNext()) { //다음 데이터값이 없을때까지 돌린다.
Map.Entry<String, String> e=
(Map.Entry<String, String>)iter.next();
System.out.println("key: "+e.getKey()+", value: "+e.getValue());//순서가 없어서 누가먼저 출력될지는 모름
}
key: 1, value: NC
key: 3, value: 키움
//이름, 점수를 한쌍으로 한 Map 자료구조를 구현하자.
//김자바 : 100 박자바 : 70
//시험응시자(key) => keySet()
//점수(value) => values() : Collection안에 있는 메소드를 잘 사용하기(iterator메소드)
//총점과 평균, 최고점수, 최저점수
Map<String, Integer> map =
new HashMap<String, Integer>();
map.put("김자바",100);
map.put("박자바", 70);
map.put("이자바", 60);
map.put("시자바", 90);
//key값 추출
Set<String> set = map.keySet(); //key를 쓰고싶으면 Set으로 가져오기
System.out.println("시험명단 : " +set);
//value값 추출
Collection<Integer> values = map.values(); //values를 쓰고싶으면 Collection으로 자동변환됨
Iterator<Integer> iter = values.iterator(); //Collection 안의 Iterator값으로 가져올 수 있음.
int total = 0;
while(iter.hasNext()) { //다음 데이터값이 없을때까지 돌린다.
int num = iter.next();
total += num;
System.out.println("총점 : "+total);
System.out.println("평균 : "+total/map.size());
System.out.println("최고점수 : "+Collections.max(values));
System.out.println("최저점수 : "+Collections.min(values));
System.out.println();
}
**hashmap 코드 -> 주소찾는것 Kosta.api => Test 참조
String reg = "([가-힣]+( |시|도)[가-힣]+(시|군|구))";
//~시에 살거나 서울, 인천, 대구, 광주, 부산, 울산 의 주소를 가지고 뒤쪽에 시, 군,구 로 끝나는 부분을
Map<String, Integer> map = new HashMap<String, Integer>();
//키워드와 갯수를 가지는 리스트에
String[] addresses = {
"서울특별시 중랑구 공릉로 13길 27", "서울특별시 중랑구 겸재로 68 (면목동)",
"서울특별시 노원구 공릉로 95 (공릉동)",
"서울특별시 구로구 가마산로 77 (구로동)", "서울특별시 마포구 가양대로 1 (상암동)",
"충청남도 천안시 동남구 성남면 5산단1로 22",
"부산광역시 동구 고관로 5 (초량동)", "인천광역시 남동구 간석로 2 (간석동)",
"충청남도 예산군 신암면 오신로 852-2", "충청남도 당진시 우강면 박원로 138",
"부산광역시 동구 중앙대로 243-13 (초량동)", "경기도 구리시 동구릉로136번길 47 (인창동)",
"서울 중랑구 공릉로 13길 27" };
Pattern p = Pattern.compile(reg);
for (String address : addresses) {
Matcher m = p.matcher(address);
if (m.find())
map.put(m.group(), map.getOrDefault(m.group(), 0) + 1);
}
for (Entry<String, Integer> s : map.entrySet())
System.out.println(s.getKey() + "에 사는 사람은 " + s.getValue() + "명");
Mission. 식당 주문관리 프로그램 (큐구조)
1. 주문요청 2. 주문처리 3. 매출액 총액 4. 종료
입력 1>Food(foodName, price)
Order(Food, amount)생성 => 자료구조 추가 //연관관계
입력 2> 자료구조에 있는 주문처리(주문된 내용 출력)
//order하나 생성. 주문처리를 큐구조로 하기 linkedlist사용
1. 주문요청하면 큐자료구조에 입력
2. 큐에서 빠져나감
입력 3> 주문처리된 메뉴에 대한 합계출력
Kosta.queue
//내가 짜다가 안돼서 포기한 코드 ->이렇게 하면 작동안함.
private Food food[] = new Food[100]; //객체 초기화만 해놓지말고 꼭 생성까지 해놓기!!! 안그럼 오류뜸
public void request() {
System.out.println("요리와 가격을 선택해주세요.");
String foodName = sc.nextLine();
int price = sc.nextInt();
int count = 0;
food[count++] = new Food(foodName, price);
for(int i=0;i<count;i++) {
queue.offer(food[i]);
}
}
public void deal() {
while(!queue.isEmpty()) {
Food choose = queue.poll();
System.out.println(choose);
}
}
public void total() {
int sum = 0;
for(int i=0;i<queue.size();i++) {
sum += food[i].getPrice();
}
System.out.println("bill : " + sum );
}
-Food
package Kosta.queue;
public class Food {
private String foodName;
private int price;
public Food() {}
public Food(String foodName, int price) {
super();
this.foodName = foodName;
this.price = price;
}
public String getFoodName() {
return foodName;
}
public void setFoodName(String foodName) {
this.foodName = foodName;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
}
-Order
package Kosta.queue;
import java.util.LinkedList;
import java.util.Scanner;
public class Order {
private Food food; //객체 초기화만 해놓지말고 꼭 생성까지 해놓기!!! 안그럼 오류뜸
private int amount;
private int total;
public Order() {}
public Order(Food food, int amount) {
super();
this.food = food;
this.amount = amount;
this.total = food.getPrice()*amount;
}
// LinkedList<Food> list = new LinkedList<Food>();
public void show() {
System.out.println("요리 : "+food.getFoodName());
System.out.println("가격 : "+food.getPrice());
System.out.println("수량 : "+amount);
}
public Food getFood() {
return food;
}
public void setFood(Food food) {
this.food = food;
}
public int getAmount() {
return amount;
}
public void setAmount(int amount) {
this.amount = amount;
}
public int getTotal() {
return this.total;
}
public void setTotal(int total) {
this.total = total;
}
}
-QueueMission(main)
package Kosta.queue;
import java.util.LinkedList;
import java.util.Scanner;
public class QueueMission {
static int totalSales = 0;
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
LinkedList<Order> list = new LinkedList<Order>();
int idx = -1;
while(true) {
System.out.println("1. 주문요청 2. 주문처리 3. 매출액 총액 4. 종료");
System.out.print("선택: ");
String menu = sc.nextLine();
switch (menu) {
case "1":
addMenu(list);
System.out.println("주문이 추가 되었습니다.");
break;
case "2":
menuService(list);
break;
case "3":
System.out.println("총매출액: " + totalSales);
break;
case "4":
System.out.println("종료");
return;
}
}
}
public static void addMenu(LinkedList<Order> list) {
Scanner sc = new Scanner(System.in);
System.out.print("요리 : ");
String foodName = sc.nextLine();
System.out.print("가격 : ");
int price = Integer.parseInt(sc.nextLine()); //문자열을 정수로 변환해줌
System.out.print("수량 : ");
int amount = Integer.parseInt(sc.nextLine());
// Food food = new Food(foodName, price);
// Order order = new Order(food, amount);
list.offer(new Order(new Food(foodName, price),amount));//위에 두개를 하나로 간결하게 표현한 것
}
public static void menuService(LinkedList<Order> list) { //큐를 구성하는 것 - LinkedList
if(!list.isEmpty()) { //if와 while의 차이는 if는 하나씩 처리하고 while은 전체 한꺼번에 처리
Order order = list.poll();
order.show();
totalSales += order.getTotal();
}
}
}
그 인터페이스를 구현해서 그 안에있는 추상메소드를 오버라이딩 하자 -> 그러면 최초의 그 방법을 사용할 수 있음
**정렬
Kosta.sort
SortExam
package Kosta.sort;
import java.util.Comparator;
import java.util.TreeSet;
import java.util.Random;
public class SortExam {
public static void main(String[] args) {
//최초의 정렬 : Comparable -> compareTo()
//정렬기준 변경 : Comparator => compare()
Random r = new Random();
TreeSet<Integer> set =
new TreeSet<Integer>(new Desending());//인터페이스 형변환 가능
for(int i=0; set.size()<6;i++) {
int num = r.nextInt(45)+1;
set.add(num);
}
System.out.println(set);
}
}
Desending
package Kosta.sort;
import java.util.Comparator;
public class Desending implements Comparator<Integer>{
@Override
public int compare(Integer o1, Integer o2) {
//내림차순에 대한 정렬기준을 정의
if(o1<o2) {
return 1; //자리를 바꾸세요
}else if(o1>o2) {
return -1; //자리 바꾸지마
}
return 0;
}
}
Person
package Kosta.sort;
public class Person implements Comparable<Person>{
private String name;
private int age;
public Person() {}
public Person(String name, int age) {
super();
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@Override
public String toString() {
return "Person [name=" + name + ", age=" + age + "]";
}
@Override
public int compareTo(Person p) { ----최초의 정렬
//나이를 기준으로 오름차순 정렬을 해보시오.
if(age < p.age) {
return -1;
}else if(age>p.age) {
return 1;
}
return 0;
}
}
SortExam2
package Kosta.sort;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class SortExam2 {
public static void main(String[] args) {
List<Person> list = new ArrayList<Person>();
list.add(new Person("김자바",23));
list.add(new Person("박자바",99));
list.add(new Person("권자바",54));
list.add(new Person("은자바",65));
list.add(new Person("이자바",88));
Collections.sort(list); //최초의 정렬기준을 안세워서 오류뜸
System.out.println(list);
}
}
"문자열".compareTo(문자열) => 음수가 나온다 =>aaa bbb 앞의 값이 더 작음
integer값을 비교하려면 a>b가 가능한데에 비해 String이면 name.compareTo(p.name)>0 이런식으로 써야함.
Collections.sort(list, new Comparator<Person>() { //파라미터가 하나 더 늘었다. -> 정렬기준을 바꾸고 싶다. =>>>>>추상클래스 : 내부클래스 선언해줌 - 한번만 다시 이용할 것이기 때문.
@Override
public int compare(Person o1, Person o2) {
//이름을 기준으로 오름차순
if(o1.getName().compareTo(o2.getName())>0) {
return 1;
}else if(o1.getName().compareTo(o2.getName())<0) {
return -1;
}
return 0;
}//익명 내부클래스 만들기~
}); //최초의 정렬기준을 안세워서 오류뜸
System.out.println(list);
[Person [name=권자바, age=54], Person [name=김자바, age=23], Person [name=박자바, age=99], Person [name=은자바, age=65], Person [name=이자바, age=88]]
☆과제
[1]phoneinfo 객체배열 ->arraylist로 바꾸기 : 1번이 우선순위임
[2]
-
정렬로 바꾸기 이름을 기준으로 1.오름차순 2.내림차순
-
종료
★★오늘해야할일
Kosta.queue (복습&코드완성) ,phone 자료구조과제
-> 되긴하는데 왜 Main에 m.addPhoneinfo(null); 이렇게 값을 넣는 걸까 초기화하는걸까?
Kosta.sort (복습) , phone 정렬과제
'FULLSTACK > JAVA' 카테고리의 다른 글
JAVA 16차시 - File, Thread (0) | 2020.11.12 |
---|---|
JAVA 15차시 - 복습, 입출력(3가지 카테고리&파일클래스) (0) | 2020.11.12 |
JAVA 13차시 - (자바API) 정규 표현식, 자료구조(ArrayList vs LinkedList) (0) | 2020.11.12 |
Java 주말과제 및 복습데이 - Calendar클래스 달력출력, 딱지문제 (0) | 2020.11.12 |
JAVA 12차시 - 객체복습, Object 클래스(toString메소드, equals메소드), Wrapper클래스, 박싱언박싱, 네스티드클래스와 네스티드 인터페이스), 자바API(String, StringBuffer, Calendar클래스) (0) | 2020.11.12 |